Welcome to my next work update for my work on 310Games’ The Krilling! This week my main goal was fixing any bugs that slipped through previously and some other minor changes. It was a lighter week, but essential fixes for the game to look polished.
My first minor change is placing the balloon possessable into the scene and ensuring it works with my new system from last week! The balloon was implemented by someone else on my team, but the placement was handed to me to reduce the number of people making scene changes and because of my new system from last week with the new HUD. I placed the balloon and added it to the serialized field of the Campfire quadrant, but the main issue was that the idea was to have multiple balloons around since it is a one time use object (it pops and gets deleted). So, when I possessed multiple different balloons, the dictionary system from last week would add each one as a different possessable!
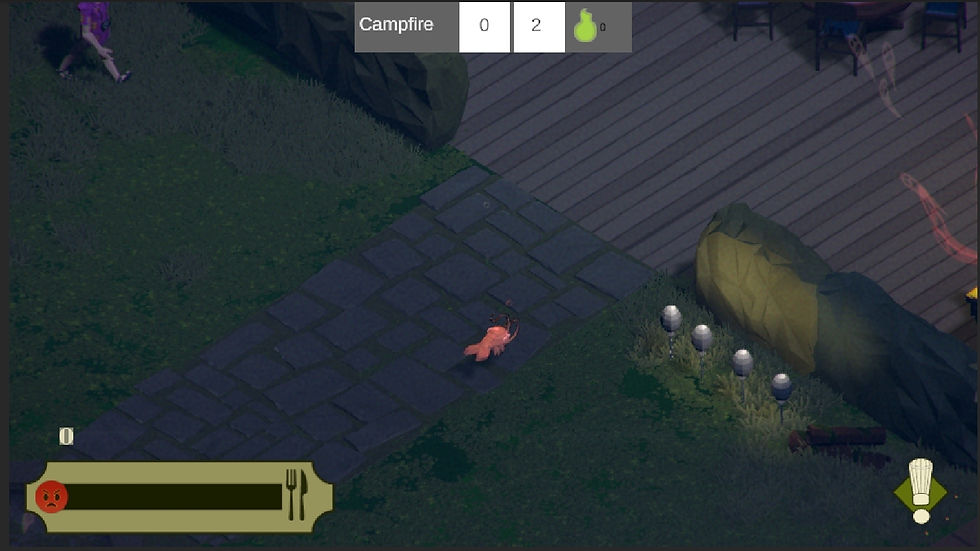
The way I fixed this issue was by storing in the dictionary the name of the possessable instead of the Possessable object! This should work the same for all other possessables, and also account for the case where there are multiple of the same kind. You can see in this following image that I have possessed and popped two of the balloons, but the tally has only gone up once.

My next minor change comes due to the new HUD. You will notice in the previous screenshots that there is a score counter above the HUD on the bottom left as well as a score counter on the new HUD on the top. We don’t need both, so I am getting rid of the one above the left HUD! We also have a score pop up that currently shows in the middle of the screen and for now I will be moving it to be underneath where the current score is now shown.
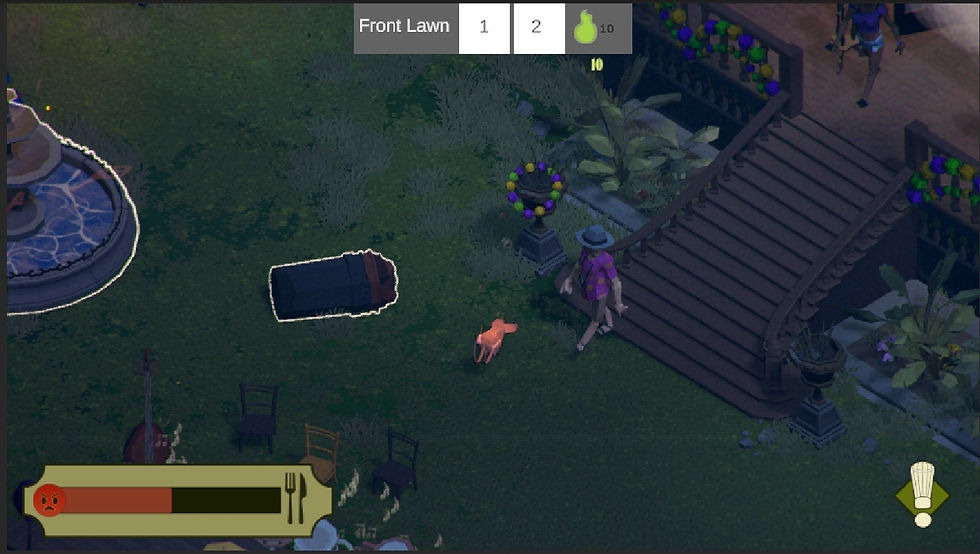
There will likely still be some changes made to the score pop up, but for now it made the most sense for it to show up next to the number it was adding to.
The next fix was on the scenario menu UI. Somehow, through testing the functionality I missed the fact that the strikethrough on completion of the scenarios only affected 1 of the text objects in the instances where there were multiple in a scenario.
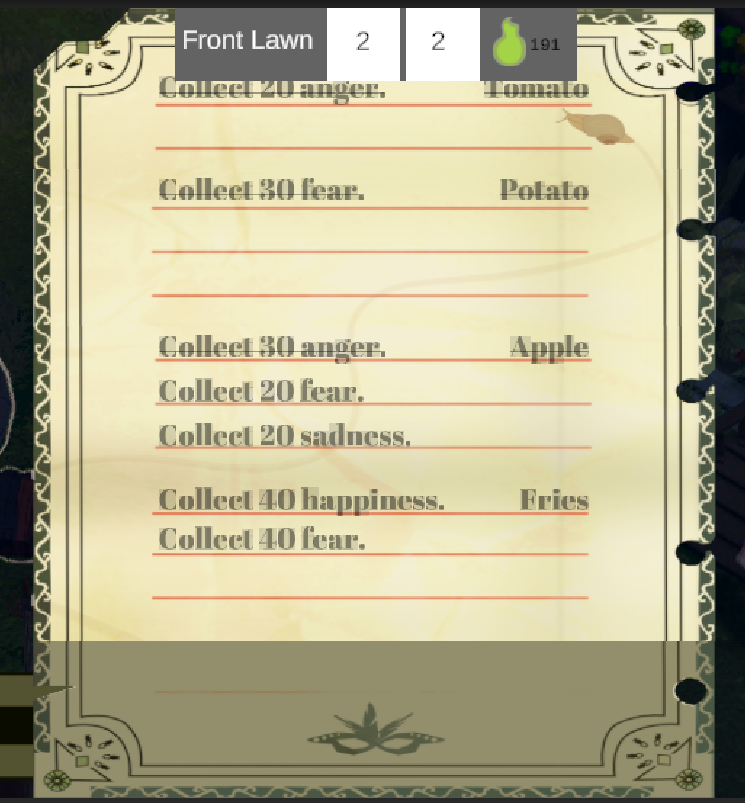
As you can see, the third scenario’s text only has the first line struck through (you can also see an additional issue with the new HUD appearing on top of the scenario menu which I will be fixing next). To fix this issue I first revisited the code I wrote that tracked these text objects and applied the strikethrough on completion of the scenario.
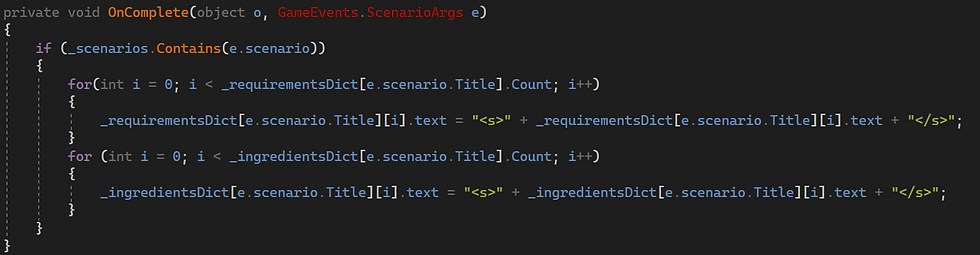
Reviewing this code did not reveal any discrepancies. The loop is simple and should be working. I figured the list must not be getting created correctly so that is where I looked next.
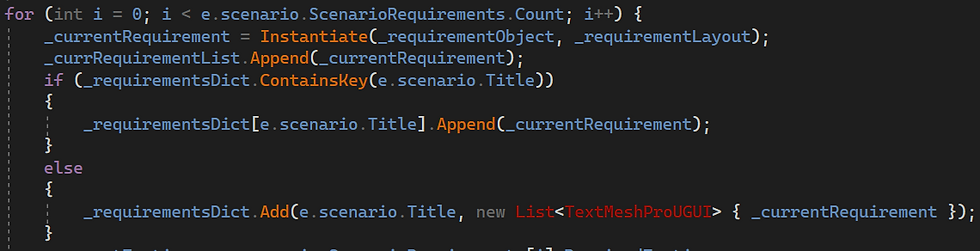
This is where the requirement list gets created, specifically the objects get added where it says “.Append”. Last week, when I was adding things to a list within a dictionary I used “.Add”. To be honest, I am not sure why, it was just the first method that came to mind when I was coding. Just for fun, I decided to see if that was the issue, and changed the code very simply to this:
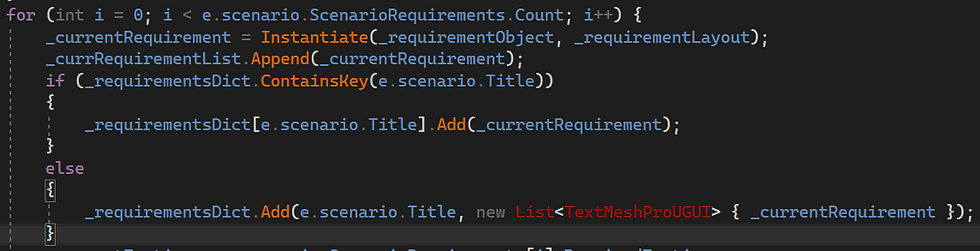
And…
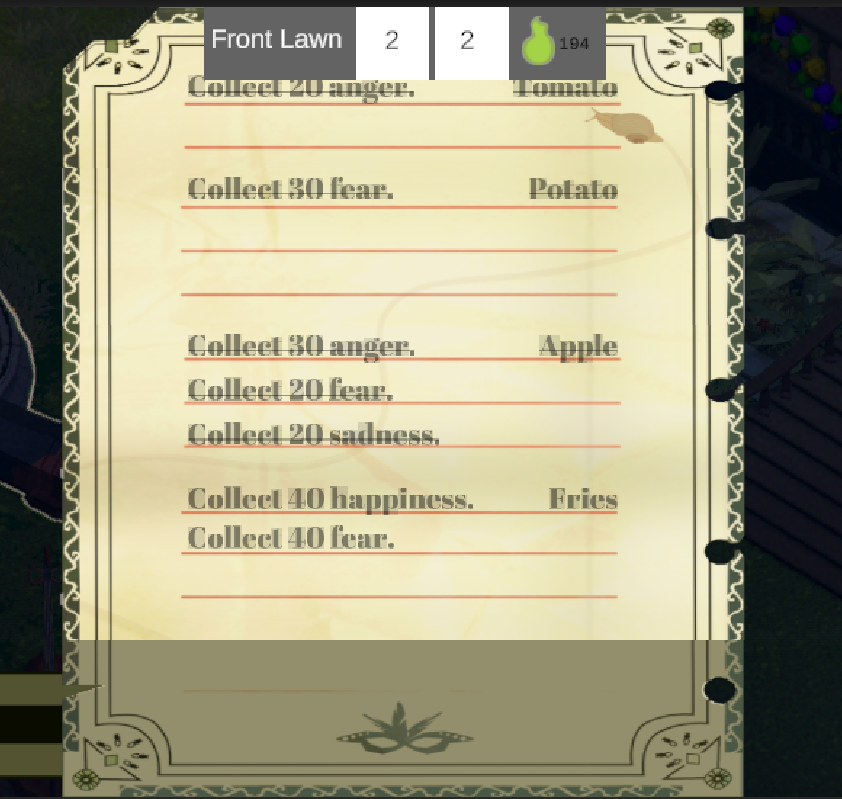
It worked! So, it appears the issue was that the list was not properly being built and thus could not be iterated through in the way I was doing it. Problem solved!
Next, as previously mentioned (and shown), the new HUD is currently displayed on top of the scenario menu. That is not something we want to occur. The problem with Unity is that as far as I could find on the internet, there is no Z ordering I can do with the UI elements. The only fix I have found so far (and I have used this for all of the UI concerning this issue) is to make the objects that need to appear on top the children of objects that need to display on the bottom. It is a strange and frustrating way to address the issue, but as far as I can find it is the only way to do it. It works because of the order in which Unity renders Children vs Parent objects. Here is the new scene hierarchy:

And here is the new HUD working with both the pause menu and the scenario menu (you can tell it is working with the pause menu because it gets slightly grayed out):
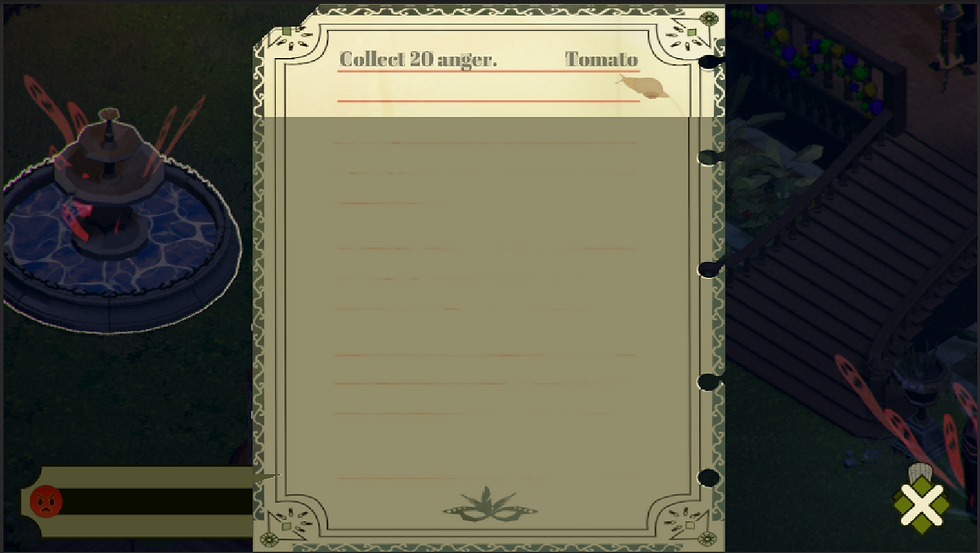
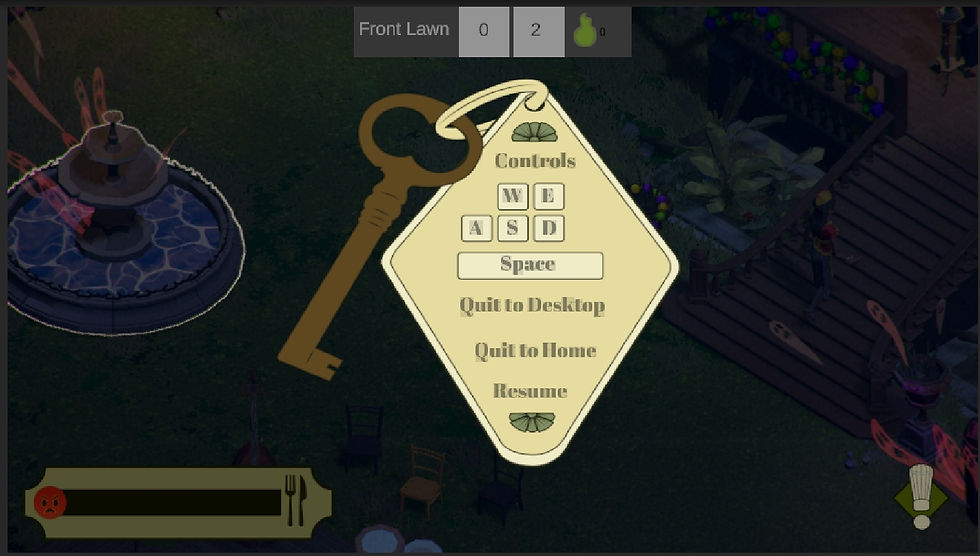
The next minor implementation I did this week was a system for notifying the player when they actually finish a scenario/gain an ingredient. As of right now the ingredients aren’t really “anything”. They are pretty much just text that appears on the screen. However, they are attached to the acquisition of new areas of the map which is tracked on the scenarios and will be how I make this pop up appear.
I started by making a quick prefab like every other UI element. It is simply a panel (for proper screen fitting and scaling) and an image component which I will be enabling and disabling.
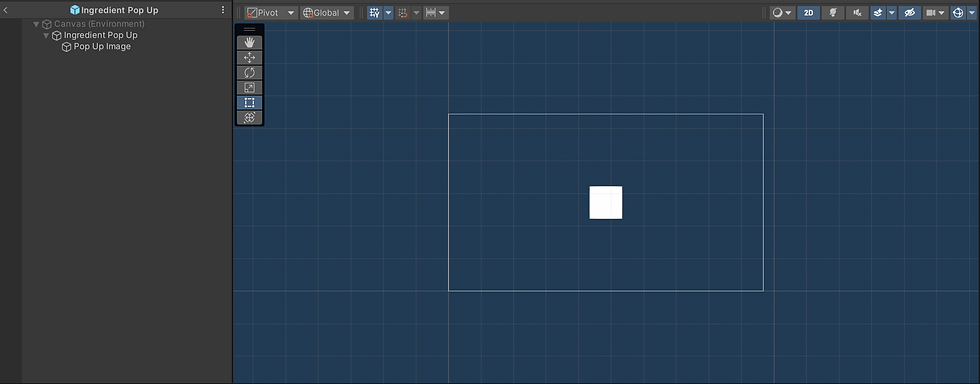
I put a script onto the panel parent object and then wrote some quick code to accept a serialized list of sprites (so we can put in each of the images we would like to pop up when a specific scenario is complete) and track a timer to make sure that the image does not stick around forever. Here is that code:
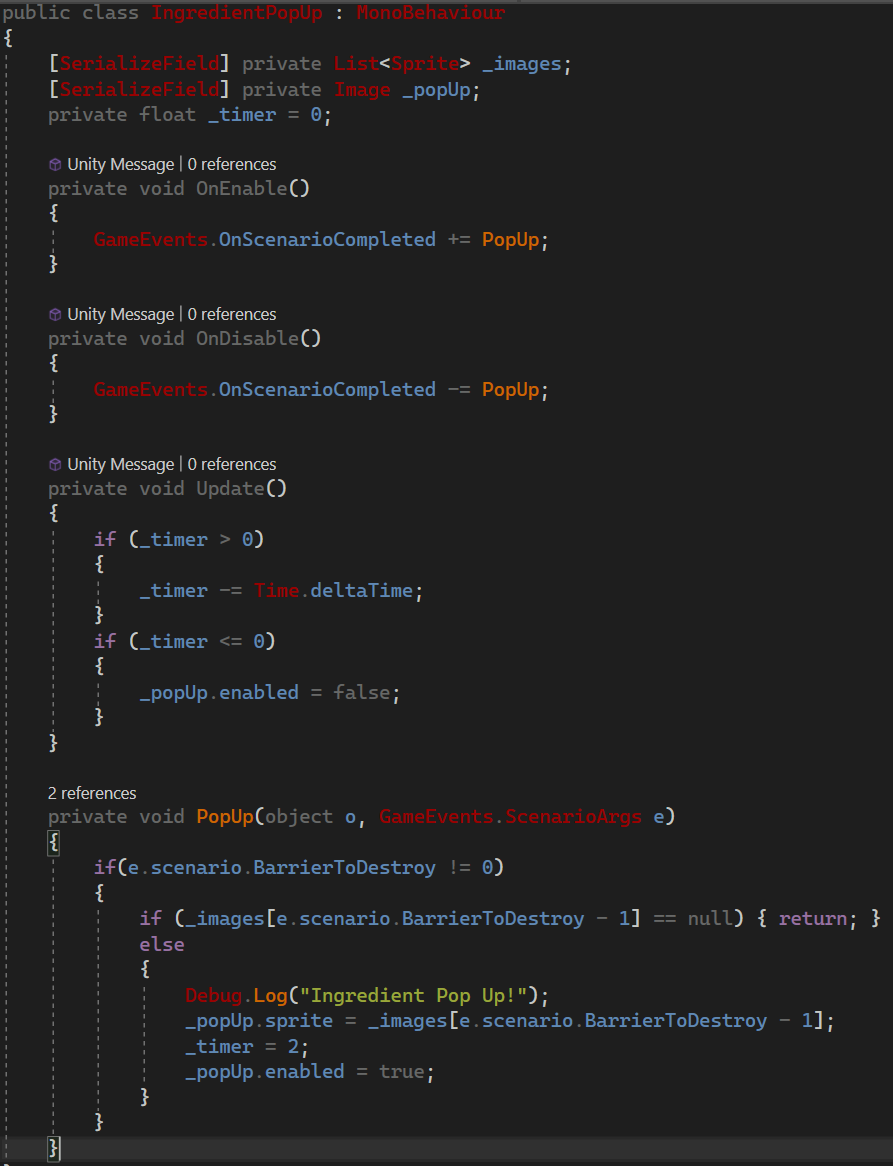
And here is what happens when a scenario is complete!
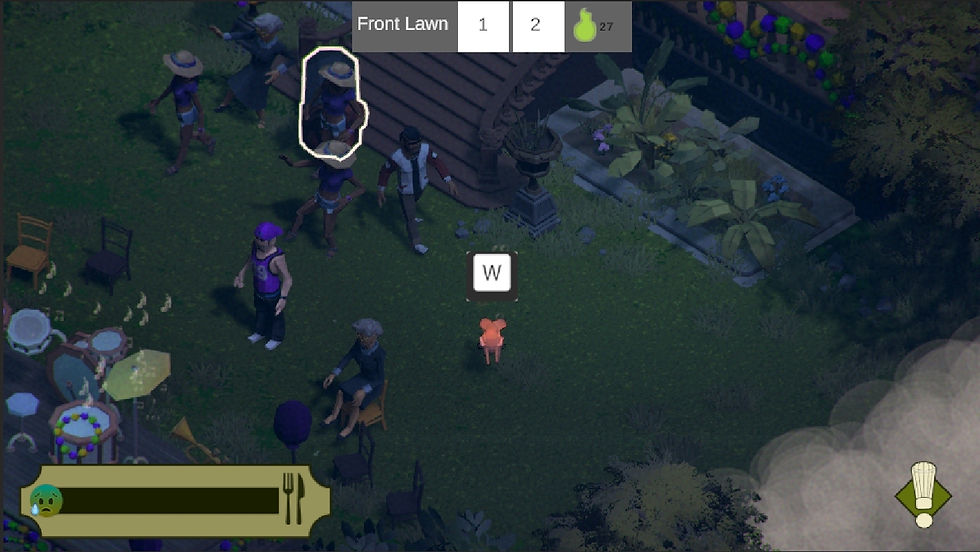
Obviously right now this image will not be what is displayed in the final iteration of this system, but until the art is made for it, this is the placeholder. The size may also change, which is easy enough to do within the prefab.
As one more change, we realized that the fog wall pictured in the above image in the bottom right was not disappearing when the player finished the second to last scenario. After that scenario we wanted the player to have full reign over the map, so that needed to be fixed. Luckily, that change was easy to do as the identifier for when the wall would be destroyed is a serialized field on the object.
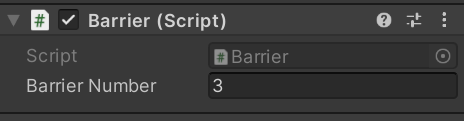
I simply changed what used to be a 4 to a 3 as shown here and it is good to go!